Exploring Google Maps API — Flutter (Part 1)
Its all about the API keys. To use google maps in your flutter app first of all we need to configure our app with Google Cloud Platform and get the API key for our app.

Here is a complete setup of how to create an API key and add the necessary package in our flutter project to get started:
SETUP
First of all add the latest version of google_maps_flutter plugin from pub.dev in pubspec.yaml file:

The next step is to get an API key from Google Cloud Platforms, whose steps are given as:
1. Go to console.cloud.google.com and create a new Project.

2. In the left drawer and APIs & Services Section open credentials:
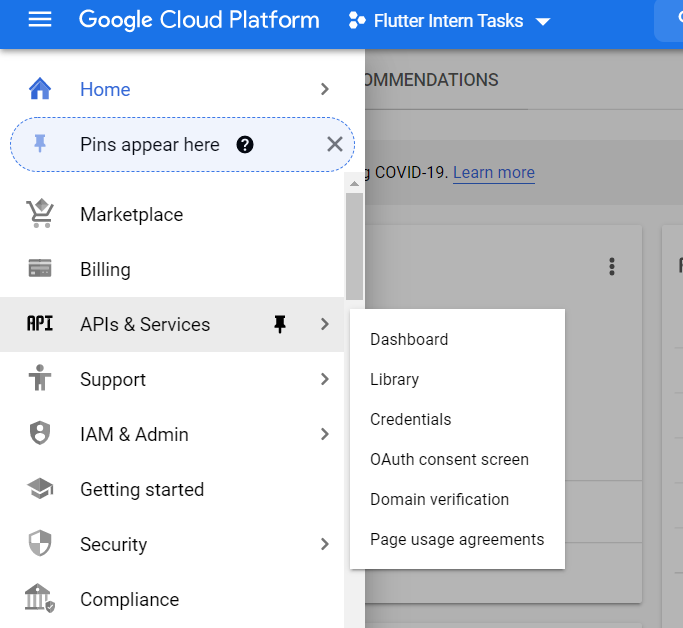
3. Click on the + Create Credentials and select API key to generate and API key.

4. In the Dashboard now you have to enable the APIs and services that you want to you use, which in our case is Maps SDK for Android:

5. Enable Maps SDK for Android.

6. Last step for setting up google maps for a flutter app is to go to your AndroidManifest.xml file (android>app>src>main>AndroidManifest.xml) and copy the following meta-data code snippet inside the <application> tag as:

Now we are good to go.

Using GoogleMap Widget
- Make Sure to import google_maps_flutter plugin.
- This provides us with a GoogleMap Widget which displays the map inside it.
- Select dummy values of latitude and longitude and assign them to variable of type LatLng.
- initialCameraPosition tells that which part of the world you want to display when the map is loaded on screen for the first time.
import 'package:flutter/material.dart';
import 'package:google_maps_flutter/google_maps_flutter.dart';class FlutterMaps extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}class _MyAppState extends State<FlutterMaps> {
final LatLng target = LatLng(30.3753, 69.3451);@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Stack(
children: [
GoogleMap(
initialCameraPosition: CameraPosition(
target: target,
zoom: 6,
),
),
],
),
),
);
}
}
Important Points to keep in mind
- initialCameraPosition is a required parameter for the GoogleMap widget. It tells that which part of the world we want to display as our map launches for the first time
- target is a parameter of CameraPostion class which takes the values of LatLng variable that we initialized earlier.
- zoom, as the name suggests it allows to set default zoomed position for our map.

You can drag, pinch to zoom in and zoom out but we are nowhere near anything useful with our maps.
So What can we do with our map?
ADDING MARKERS ON A MAP:
Let’s start with adding a couple of marker on our map. Some default LatLng values and some markers by tapping anywhere on the map.
- Create a list of markers to display on the map as some default values:

- In the GoogleMap widget, there is property called markers set the markers property to display markers listed in myMarkers:
GoogleMap(.....markers: Set.from(myMarkers),),
Result:

- Adding markers using onTap property of our GoogleMap Widget. Create a function called _handleTap with the positional parameter of type LatLng. _handleTap function will give us the values of latitude and longitude where ever we tap on the map and in the setState we add those values in our myMarkers list.
_handleTap(LatLng position) {setState(() {myMarkers.add(Marker(markerId: MarkerId(position.toString(),),position: position),);});}
And in GoogleMap widget set the onTap property to _handleTap function.
GoogleMap(onTap: _handleTap,
........ // rest of the code remains the same),
